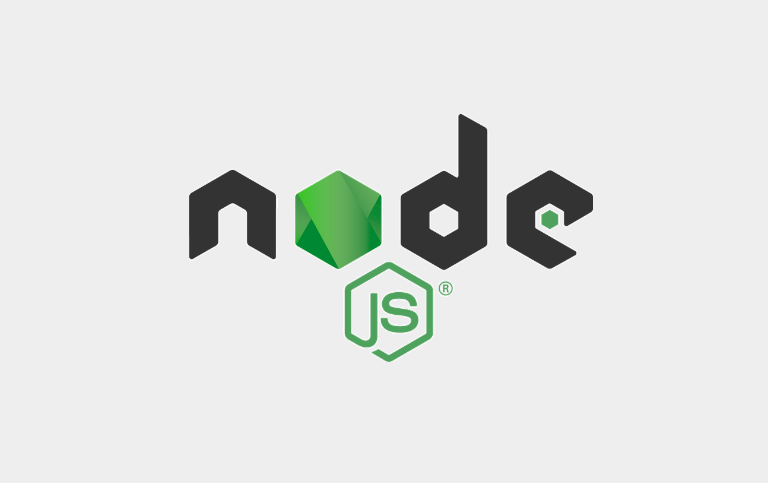
投稿日:2024/12/29 最終更新日:2024/12/29
個人的なNode.jsについてのまとめ
Node.jsでシンプルなサーバーを作成
"use strict";
const port = 3000;
const http = require("http");
const fs = require("fs");
function readFile(file, res) {
fs.readFile(`./${file}`, (err, data) => {
if(err) {
console.error("Not reading file");
};
res.end(data);
});
};
const app = http.createServer((req, res) => {
if(req.url === "/" && req.method === "GET") {
res.writeHead(200, {
"Content-type": "text/html",
});
readFile("view/index.html", res);
} else if(req.url === "/public/image/nodejs.png" && req.method === "GET") {
res.writeHead(200, {
"Content-type": "image/png",
});
readFile("public/image/nodejs.png", res);
} else if(req.url === "/public/css/style.css" && req.method === "GET") {
res.writeHead(200, {
"Content-Type": "text/css",
});
readFile("public/css/style.css", res);
} else {
res.writeHead(404, {
"Content-Type": "text/html",
});
res.end(`Not found : ${res.url}`);
console.log();
};
});
app.listen(port);
console.log(`The server has started and is listening on port number: ${port}`);
fsモジュール
Node.jsでファイルの読み書きを行うための関数提供用モジュールであり、fsモジュールは同期形式と非同期形式の両方が提供されている。
標準モジュールではnode:fs
のようにnode:
プリフィックスをつけてインポートすることも可能。
import { readFile } from "node:fs";
非同期APIはfs/promises
というモジュール名で参照できる。
import { readFile } from "node:fs/promises";
※サードパーティー製のモジュールと区別するためにnode:
プリフィックスは推奨
fs/promises
モジュールはPromiseを返し、非同期処理が成功した場合は返ってきたPromise
インスタンスがresolveされ、失敗した場合はrejectされる。
import fs from "node:fs/promises";
fs.readFile("sample.js").then(data => {
// resolveされた場合
console.log(data);
}).catch(err => {
// rejectされた場合
console.error(err);
});
Node.jsはシングルスレッドであり、他処理をブロックしにくい非同期形式のAPIを選ぶことが多く、fs/promises
モジュール以外に非同期APIがたくさんあることは留意すること。
readFile関数
readFile(path[, options])
関数はファイルを読み込むための関数。
第1引数には返すファイルを指定、第2引数には文字コードなどのオプションを指定する。
非同期処理を行いたい場合はreadFileSync(path[, options])
を使用する。
createServer関数
createServer
関数はhttp
モジュールで使用できる関数であり、ヘッダー情報や表示コンテンツの設定を行うことでサーバー処理を作成できる。
const http = require('http');
const port = 3000;
const app = http.createServer(function(req, res) {
// サーバー処理
})
app.listen(port);
req
ではURL情報を取得できるためリクエストごとに指定のファイル表示を行うことができる。
また、res
はresponseオブジェクトのメソッドであり、ヘッダー情報をレスポンスに書き出すことなどができる。
なので、サンプルではres.writeHead(200, {"Content-type": "image/png"});
を作成しており、ステータスコードは200で表示コンテンツは画像形式を指定するなどを設定している。
res.end()
はHTTPレスポンスのプロセスを終了する関数であり、正常終了を行うために必要な関数となる。
最後にlisten(port)
を使用することで指定ポートでのサーバー待ち受け開始することができる。
※listen(0)
とするとランダムポートを取得してくれる
Expressで同じ処理のサーバーを作る
const port = 3000;
const express = require("express");
const app = express();
app.use(express.static("static"));
app.get("/", (req, res) => {
res.writeHead(200);
res.end();
});
app.listen(port);
express
はnpmパッケージをインストールして利用するフレームワーク。
利用はconst app = express();
のように簡単に始められる。
先ほどとの違いとしてはapp.use(express.static("static"));
のような指定をすることで静的ファイルをフォルダーごと一式読み込むことができる。
そのためapp.get("/", (req, res) => {
の処理は分岐処理をする必要がなく、パスに対して自動的にファイルを返してくれる。